owned this note
owned this note
Published
Linked with GitHub
<!-- .slide: data-background="https://i.imgur.com/kiAxo5r.png" -->
## r2 and RE
---
### whoami
```shell
$ HANDLE=sudhackar
$ whereis $HANDLE
Payatu Technologies
$ man $HANDLE
RE, Fuzzing and Exploit Dev shenanigans
$ info $HANDLE
CTFs with RE/pwn/Crypto @ ByteBandits, IITI
```
---
<!-- .slide: data-background="https://i.imgur.com/kiAxo5r.png" -->
### radare2
* https://github.com/radare/radare2
* Archs : i386, x86-64, ARM, MIPS, PowerPC, SPARC ...
* Files : ELF, Mach-O, PE, PE+, MZ, COFF ...
* Platforms : win, linux, osx, android, iOS ...
* Bindings : py, lua, go, ruby ...
---
<!-- .slide: data-background="https://i.imgur.com/kiAxo5r.png" -->
### installation
```sh
$ sys/user.sh
```
* Always use r2 from git
* utilities: rax2, rabin2, rasm2, radiff2, rafind2, rahash2, radare2, rarun2 ...
* base conversion, bin info, asm, bindiff, search/edit, hash, re, run ...
---
### why?
* purely command line
* Cutter : A Qt and C++ GUI for radare2 reverse engineering framework
* free as in freedom
* it's cool
---

---
### Usability
---
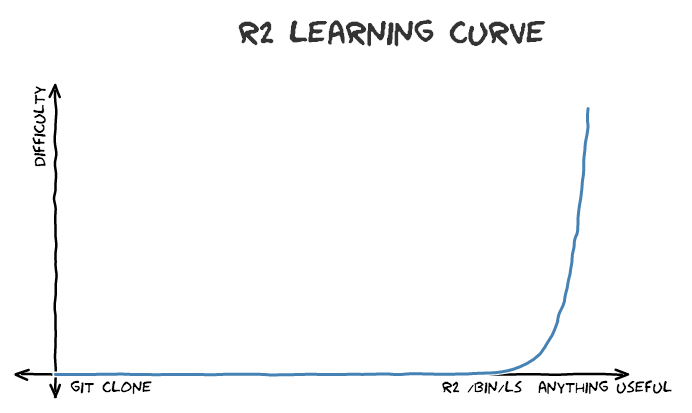
---
<!-- .slide: data-background-video="https://video.twimg.com/tweet_video/C1wATZQWQAIIKED.mp4" -->
---
Radare is a LGPL portable reversing framework that can:
* Debug natively or use remote targets (gdb, r2pipe, winedbg, windbg)
* Perform forensics on filesystems and data carving
* Visualize data structures of several file types
* Patch programs to uncover new features or fix vulnerabilities
* Use powerful analysis capabilities to speed up reversing
* Aid in software exploitation
---
### Reverse Engineering
---
### you should start with this
* executable : ELF, PE, APK/dex?
* low level code : asm, IL/VMs?
* basic blocks
* control flow
---
### hello.c
```c
#include <stdio.h>
int main(int argc, char **argv)
{
puts("Hello World!");
return 0;
}
```
---
### hello r2
```shell
$ r2 hello-x86_64
-- Now with more better English!
[0x00400400]> ?
Usage: [.][times][cmd][~grep][@[@iter]addr!size][|>pipe] ; ...
Append '?' to any char command to get detailed help
```
* Learn to `?`
---
### analysis
```shell
[0x00400400]> ?~anal
| a[?] analysis commands
[0x00400400]> a~:1
| aa[?] analyze all (fcns + bbs) (aa0 to avoid sub renaming)
[0x00400400]> a?~:1..4
| aa[?] analyze all (fcns + bbs) (aa0 to avoid sub renaming)
| a8 [hexpairs] analyze bytes
| ab[b] [addr] analyze block at given address
```
---
### analysis
```shell
[0x00400400]> aa
[x] Analyze all flags starting with sym. and entry0 (aa)
[0x00400400]> afl
....
0x004004e0 1 7 entry1.init
0x004004e7 1 32 sym.main
....
[0x00400400]> afl?
Usage: afl List all functions
| afl list functions
| afl+ display sum all function sizes
| aflc count of functions
| aflj list functions in json
| afll list functions in verbose mode
| afllj list functions in verbose mode (alias to aflj)
| aflq list functions in quiet mode
| aflqj list functions in json quiet mode
| afls sort function list by address
[0x00400400]>
```
---
### r2 hello
```shell
[0x00400400]> s?
Usage: s # Help for the seek commands. See ?$? to see all variables
| s Print current address
| s:pad Print current address with N padded zeros (defaults to 8)
| s addr Seek to address
```
----
```shell
$ r2 hello-x86_64
-- Default scripting languages are NodeJS and Python.
[0x00400400]> aaa
[x] Analyze all flags starting with sym. and entry0 (aa)
[Invalid instruction (possibly truncated) at 0x4000d1
[x] Analyze function calls (aac)
[x] Analyze len bytes of instructions for references (aar)
[x] Constructing a function name for fcn.* and sym.func.* functions (aan)
[x] Type matching analysis for all functions (afta)
[x] Use -AA or aaaa to perform additional experimental analysis.
[0x00400400]>
```
----
```shell
[0x00400400]> s sym.main
[0x004004e7]> pdf
;-- main:
┌ (fcn) sym.main 32
│ sym.main (int argc, char **argv, char **envp);
│ ; var int local_10h @ rbp-0x10
│ ; var int local_4h @ rbp-0x4
│ ; arg int argc @ rdi
│ ; arg char **argv @ rsi
│ ; DATA XREF from entry0 (0x40041d)
│ 0x004004e7 55 push rbp
│ 0x004004e8 4889e5 mov rbp, rsp
│ 0x004004eb 4883ec10 sub rsp, 0x10
│ 0x004004ef 897dfc mov dword [local_4h], edi ; argc
│ 0x004004f2 488975f0 mov qword [local_10h], rsi ; argv
│ 0x004004f6 bf94054000 mov edi, str.Hello_World ; 0x400594 ; "Hello World!"
│ 0x004004fb e8f0feffff call sym.imp.puts ; int puts(const char *s)
│ 0x00400500 b800000000 mov eax, 0
│ 0x00400505 c9 leave
└ 0x00400506 c3 ret
[0x004004e7]>
````
----
* `VV` for visual mode, p/P to change, semi vim bindings

----
#### Lets Strip
```c=
// gcc -m32 -s -no-pie -fno-pic if-else.c -o if-else
#include <stdio.h>
int main(int argc, char **argv) {
if (argc == 2)
puts("two");
else
puts("yes");
return 0;
}
```
----
#### r2 strip
```shell
$ r2 -AA if-else
[x] Analyze all flags starting with sym. and entry0 (aa)
[x] Analyze function calls (aac)
[x] Analyze len bytes of instructions for references (aar)
[x] Constructing a function name for fcn.* and sym.func.* functions (aan)
[x] Enable constraint types analysis for variables
-- You are probably using an old version of r2, go checkout the git!
[0x08048310]> afl~main
0x080482f0 1 6 sym.imp.__libc_start_main
```
----
#### no main no pain
```c
int __libc_start_main(int (*main)(int, char **, char **), int argc,
char **ubp_av, void (*init)(void), void (*fini)(void),
void (*rtld_fini)(void), void(*stack_end));
```
----
```shell
$ r2 -AA if-else
[0x08048310]> s sym.imp.__libc_start_main
[0x080482f0]> axt
entry0 0x804833d [CALL] call sym.imp.__libc_start_main
[0x080482f0]> ax?
Usage: ax[?d-l*] # see also 'afx?'
| ax list refs
| ax* output radare commands
| ax addr [at] add code ref pointing to addr (from curseek)
| ax- [at] clean all refs/refs from addr
| ax-* clean all refs/refs
| axc addr [at] add generic code ref
| axC addr [at] add code call ref
| axg [addr] show xrefs graph to reach current function
| axg* [addr] show xrefs graph to given address, use .axg*;aggv
| axgj [addr] show xrefs graph to reach current function in json format
| axd addr [at] add data ref
| axq list refs in quiet/human-readable format
| axj list refs in json format
| axF [flg-glob] find data/code references of flags
| axt [addr] find data/code references to this address
| axf [addr] find data/code references from this address
| axff [addr] find data/code references from this function
| axs addr [at] add string ref
[0x080482f0]>
```
----
```shell
[0x08048310]> s entry0
[0x08048310]> pdf
;-- section..text:
;-- eip:
┌ (fcn) entry0 50
│ entry0 ();
│ 0x08048310 31ed xor ebp, ebp ; [14] -r-x section size 450 named .text
│ 0x08048312 5e pop esi
│ 0x08048313 89e1 mov ecx, esp
│ 0x08048315 83e4f0 and esp, 0xfffffff0
│ 0x08048318 50 push eax
│ 0x08048319 54 push esp
│ 0x0804831a 52 push edx
│ 0x0804831b e823000000 call fcn.08048343
│ 0x08048320 81c3e01c0000 add ebx, 0x1ce0
│ 0x08048326 8d83d0e4ffff lea eax, [ebx - 0x1b30]
│ 0x0804832c 50 push eax ; func fini
│ 0x0804832d 8d8370e4ffff lea eax, [ebx - 0x1b90]
│ 0x08048333 50 push eax ; func init
│ 0x08048334 51 push ecx ; char **ubp_av
│ 0x08048335 56 push esi ; int argc
│ 0x08048336 c7c026840408 mov eax, 0x8048426
│ 0x0804833c 50 push eax ; func main
└ 0x0804833d e8aeffffff call sym.imp.__libc_start_main ; int __libc_start_main(func main, int argc, char **ubp_av, func init, func fini, func rtld_fini, void *stack_end)
[0x08048310]> f main @ 0x8048426
[0x08048310]> s main
```
----
```shell
[0x08048426]> pdf
p: Cannot find function at 0x08048426
[0x08048426]> af
[0x08048426]> pdf
┌ (fcn) main 71
│ main (int argc, char **argv, char **envp);
│ ; var int local_4h @ ebp-0x4
│ ; arg int arg_4h @ esp+0x4
│ ; DATA XREF from entry0 (0x8048336)
│ 0x08048426 8d4c2404 lea ecx, [arg_4h] ; 4
│ 0x0804842a 83e4f0 and esp, 0xfffffff0
│ 0x0804842d ff71fc push dword [ecx - 4]
│ 0x08048430 55 push ebp
│ 0x08048431 89e5 mov ebp, esp
│ 0x08048433 51 push ecx
│ 0x08048434 83ec04 sub esp, 4
│ 0x08048437 89c8 mov eax, ecx
│ 0x08048439 833802 cmp dword [eax], 2
│ ┌─< 0x0804843c 7512 jne 0x8048450
│ │ 0x0804843e 83ec0c sub esp, 0xc
│ │ 0x08048441 68f0840408 push 0x80484f0
│ │ 0x08048446 e895feffff call sym.imp.puts ; int puts(const char *s)
│ │ 0x0804844b 83c410 add esp, 0x10
│ ┌──< 0x0804844e eb10 jmp 0x8048460
│ ││ ; CODE XREF from main (0x804843c)
│ │└─> 0x08048450 83ec0c sub esp, 0xc
│ │ 0x08048453 68f4840408 push 0x80484f4
│ │ 0x08048458 e883feffff call sym.imp.puts ; int puts(const char *s)
│ │ 0x0804845d 83c410 add esp, 0x10
│ │ ; CODE XREF from main (0x804844e)
│ └──> 0x08048460 b800000000 mov eax, 0
│ 0x08048465 8b4dfc mov ecx, dword [local_4h]
│ 0x08048468 c9 leave
│ 0x08048469 8d61fc lea esp, [ecx - 4]
└ 0x0804846c c3 ret
```
----

----
## Demo
---
### Where to learn from
* https://github.com/radare/radare2/issues
* https://radare.gitbooks.io/radare2book
* http://beta.rada.re/en/latest/
* http://www.telegram.me/radare
* https://reverseengineering.stackexchange.com/questions/tagged/radare2
* Radare Cheatsheet
---
### Way forward
* I expect to continue this series in upcoming null meets
* And keep updating these slides
---
## DANKE
----
# radare2