owned this note
owned this note
Published
Linked with GitHub
---
tags: fablab, esp32, mdef, fabacademy, fabricademy
---
# ESP32 Feather
[TOC]
:::info
A lot of the content in this document comes from the [Adafruit page](https://learn.adafruit.com/adafruit-huzzah32-esp32-feather) where you can find more details.
:::
## Specifications
* 240 MHz dual core Tensilica LX6 microcontroller with 600 DMIPS
* Integrated 520 KB SRAM
* Integrated 802.11b/g/n HT40 Wi-Fi transceiver, baseband, stack and LWIP
* Integrated dual mode Bluetooth (classic and BLE)
* 4 MByte flash
* On-board PCB antenna
* Ultra-low noise analog amplifier
* Hall sensor
* 10x capacitive touch interface
* 32 kHz crystal oscillator
* 3 x UARTs (only two are configured by default in the Feather Arduino IDE support, one UART is used for bootloading/debug)
* 3 x SPI (only one is configured by default in the Feather Arduino IDE support)
* 2 x I2C (only one is configured by default in the Feather Arduino IDE support)
* 12 x ADC input channels
* 2 x I2S Audio
* 2 x DAC
* PWM/timer input/output available on every GPIO pin
* OpenOCD debug interface with 32 kB TRAX buffer
* SDIO main/secondary 50 MHz
* SD-card interface support
[Specifications](https://espressif.com/en/products/hardware/esp32/overview) from Espressif about the ESP32 microcontroller.
## Pinout
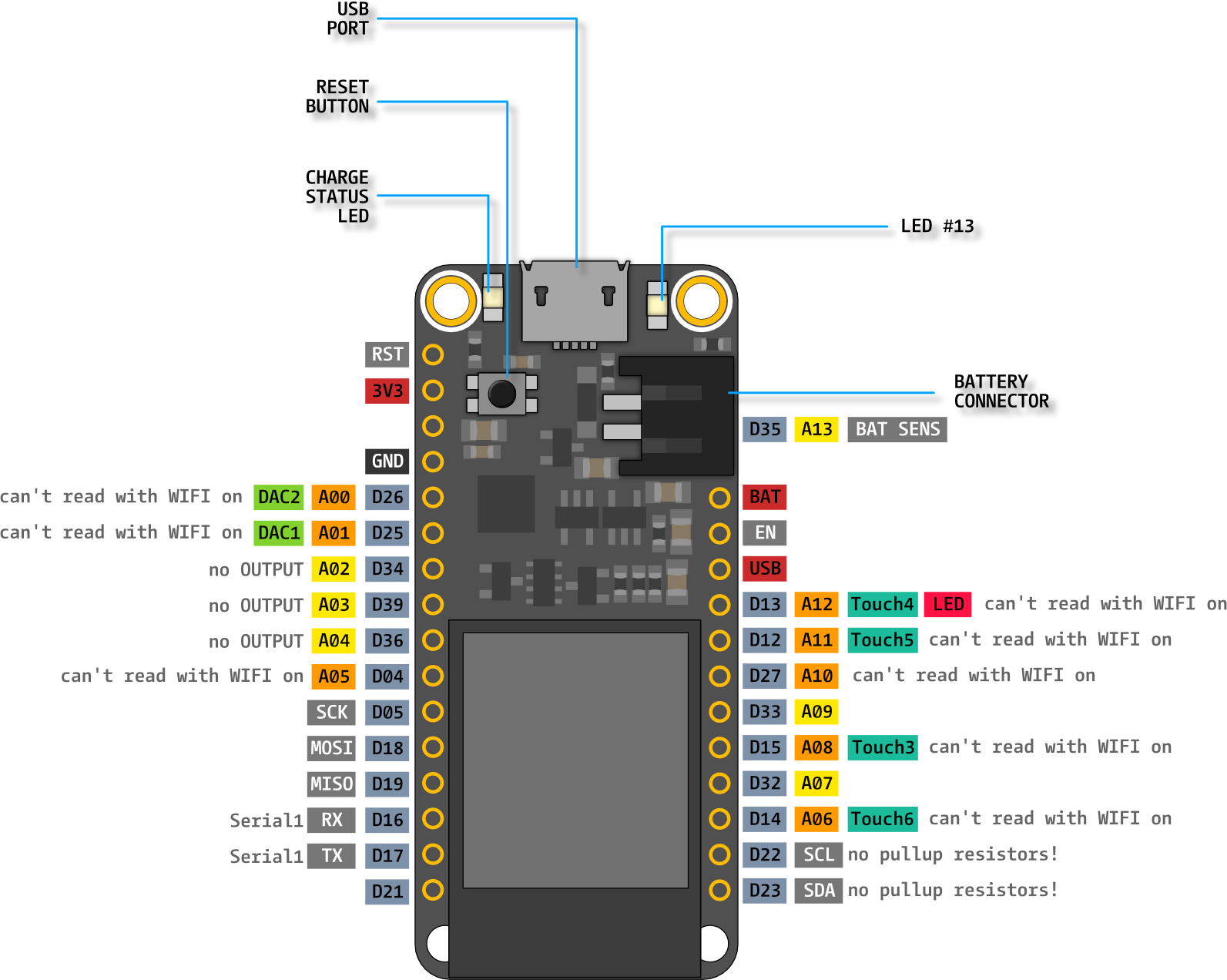
:::spoiler Click the arrow for **more images**
[](https://i.imgur.com/AVvORhx.png)


:::
## Power
You can power your board via USB cable, with a LiPo battery or with both. When the USB power is present, the feather will automatically switch over to USB for power, as well as start charging the battery (if attached).
There's also a CHG LED next to the USB jack, which will light up while the battery is charging. This **LED might also flicker if the battery is not connected, it's normal.**
To check your battery level you can read **half** of the battery voltage on pin A13. Don't forget to double the voltage you read, since there is a divider. You can find out more about what means the voltage you read [here](https://learn.adafruit.com/li-ion-and-lipoly-batteries/voltages).
**Power related pins**
**BAT** - This is the positive voltage to/from the JST jack for the optional Lipoly battery, its only powered when the battery is present!
**USB** - this is the positive voltage to/from the micro USB jack if connected, is only powered when USB is connected!
**3V** - this is the output from the 3.3V regulator. **The regulator can supply 500mA peak but half of that is drawn by the ESP32**, and it's a fairly power-hungry chip. So if you need a ton of power for stuff like LEDs, motors, etc. Use the USB or BAT pins, and an additional regulator
## Using Arduino IDE

### Install Arduino IDE
If you haven't yet go ahead and install the Arduino IDE, currently there are two main versions the **Legacy IDE (1.8.X)** and the **Arduino IDE 2.0.X**, in our experience (2022) on some situations v2 is less stable than the more tested v1.
You can find both versions for diferent OS's on arduino [downloads](https://www.arduino.cc/en/software) page.
### Serial driver
On Windows and Mac computers you need to install the SiLabs CP2104 Driver. You can download it from [here](http://www.silabs.com/products/development-tools/software/usb-to-uart-bridge-vcp-drivers). cThe driver comes in a _zip_ compressed file, be sure to extract it to a separated folder and install it as usual depending on you OS.
You will need to reopen the Arduino IDE after driver installation. And in some situations a reboot can help!
### ESP32 board definitions
To be able to program ESP32 microcontroller boards from Arduino IDE you need to install the [Arduino core](https://github.com/espressif/arduino-esp32#using-through-arduino-ide) for ESP32. I you want to know more about it you can check their [documentation](https://docs.espressif.com/projects/arduino-esp32/en/latest/getting_started.html).
First open Arduino and go to _File → Preferences_, copy the link under this text and paste it in the _Additional Boards Manager URLs_ input frame:
~~~
https://raw.githubusercontent.com/espressif/arduino-esp32/gh-pages/package_esp32_index.json
~~~
:::spoiler Version 2.0.X image

:::

Click _OK_ and go to _Tools → Boards → Boards Manager_ search for _esp32_ core and click install.
:::spoiler Version 2.0.X image

:::

### Testing the board
:::danger
Describe blink upload process with screenshots fort selecting port and board.
:::
## Input/Output
All the GPIO pins (marked with DXX on the pinout) can be used as an input or output using digitalWrite and digitalRead functions.
### [DigitalWrite](https://www.arduino.cc/reference/en/language/functions/digital-io/digitalwrite/)
```=arduino
void setup() {
pinMode(BUILTIN_LED, OUTPUT); // Initialize the BUILTIN_LED pin as an output
}
// the loop function runs over and over again forever
void loop() {
digitalWrite(BUILTIN_LED, LOW); // Turn the LED on
delay(1000); // Wait for a second
digitalWrite(BUILTIN_LED, HIGH); // Turn the LED off
delay(2000); // Wait for two seconds (to demonstrate the active low LED)
}
```
### [DigitalRead](https://www.arduino.cc/reference/en/language/functions/digital-io/digitalread/)
```=arduino
// You can try a digital input with
// a push button connected to pin D21 and ground (GND)
int pushButton = D21;
// the setup routine runs once when you press reset:
void setup() {
// initialize serial communication
Serial.begin(115200);
// make the pushbutton's pin an input and activate the internal pullup resistor:
pinMode(pushButton, INPUT_PULLUP);
}
// the loop routine runs over and over again forever:
void loop() {
// read the input pin:
int buttonState = digitalRead(pushButton);
// print out the state of the button:
Serial.println(buttonState);
delay(1); // delay in between reads for stability
}
```
### [AnalogRead](https://www.arduino.cc/reference/en/language/functions/analog-io/analogread/)
All the pins named AX (from A0 to A13) can be used as analog inputs, you can try reading your battery voltage, wich is an indicator on battery charge, reading the pin A13 (with the battery connected)
```=arduino
// the setup routine runs once when you press reset:
void setup() {
// initialize serial communication at 115200 bits per second:
Serial.begin(115200);
}
// the loop routine runs over and over again forever:
void loop() {
// read the input on analog pin A13:
int sensorValue = analogRead(A13);
// print out the value you read:
Serial.println(sensorValue);
delay(1); // delay in between reads for stability
}
```
### [AnalogWrite](https://www.arduino.cc/reference/en/language/functions/analog-io/analogwrite/)
Analog values as outputs can be achieved usin [PWM](https://en.wikipedia.org/wiki/Pulse-width_modulation) with the analogWrite() function.
```clike
int led = LED_BUILTIN; // the PWM pin the LED is attached to
int brightness = 0; // how bright the LED is
int fadeAmount = 5; // how many points to fade the LED by
// the setup routine runs once when you press reset:
void setup() {
// declare pin to be an output:
pinMode(led, OUTPUT);
}
// the loop routine runs over and over again forever:
void loop() {
// set the brightness:
analogWrite(led, brightness);
// change the brightness for next time through the loop:
brightness = brightness + fadeAmount;
// reverse the direction of the fading at the ends of the fade:
if (brightness <= 0 || brightness >= 255) {
fadeAmount = -fadeAmount;
}
// wait for 30 milliseconds to see the dimming effect
delay(30);
}
```
## [WiFi](http://www.arduino.cc/en/Reference/WiFi)
With this simple example we can try connecting to a WiFi network:
```clike
#include <Arduino.h>
#include <WiFi.h>
void setup() {
Serial.begin(115200);
WiFi.begin("ssid", "password");
while (WiFi.status() != WL_CONNECTED) {
Serial.println("Wifi connecting...");
delay(500);
}
Serial.println("Wifi connected");
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
}
void loop() {
// put your main code here, to run repeatedly:
}
```
## Library installation
Installing llibraries is done through the _Arduino library manager_ (_Sketch → Include Library → Manage libraries._)
:::spoiler Version 2.0.X image

:::

___
:::info
**References**
Arduino [Built-in Examples](https://docs.arduino.cc/built-in-examples/) documentation
Arduino [Language Reference](https://www.arduino.cc/reference/en/)
Adafruit HUZZAH32 - ESP32 Feather [Page](https://learn.adafruit.com/adafruit-huzzah32-esp32-feather/overview)
Arduino [Libraries Documentation](https://www.arduino.cc/reference/en/libraries/)
:::