# Web Hacking Quickstart Reference
## HTML
Basic build up of an HTML page:
```html
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0" >
<!-- a comment: here could be lots of more meta tags -->
<title>This is a simple placeholder page</title>
<style>
/* some style sheet declarations */
</style>
<link rel="stylesheet" href="path/to/stylefile.css">
<script src="some/path/to/a/file.js"></script>
</head>
<body>
<header>
<h1>This is a simple placeholder page</h1>
</header>
<p>
No content here really
</p>
<footer>
<hr />
<small>🄯 by nothingness 🍪</small>
</footer>
<script src="https://tantemalkah.at/2022/hack-the-heck-out-of-this-website/hello.js"></script>
</body>
</html>
```
References:
* [HTML: HyperText Markup Language](https://developer.mozilla.org/en-US/docs/Web/HTML) @ MDN Web Docs
* [HTML Element Reference](https://www.w3schools.com/tags/default.asp) and [HTML Tutorial](https://www.w3schools.com/html/default.asp) @ W3Schools
## Javascript
Here is a short language overview, in case you know other programming languages than Javascript. The following snippets are from jackies artful coding course (you can also check the slides of [session 2 starting in the JS intro section](https://tantemalkah.at/2021/artful-coding/slides/session2.html#/13)):
A bit about variables and logging:
```javascript
/*
This is a comment about some placeholder variables.
Actually, it does not say an awful lot now anyway.
*/
var myNumber = 23;
let myAnswer = 42;
const myState = "This will never change!";
// Now, let's produce some very important debug output
console.log('Hey there, what\'s up? I have something for you:');
console.log('A number:', myNumber);
console.log('THE answer:', myAnswer);
console.log(myState);
```
Data types:
```javascript
let myString = 'Francine';
let myLongerString = 'Francine is a lovely name!';
let myNumber = 5000;
let myBoolen = true;
let myOtherBoolen = false;
let myArray = [42, 'is the answer', true, myLongerString, myBoolean];
let myObject = {
name: 'Elvira',
type: 'Bat',
strength: 1000,
mana: 777,
alive: true
};
myObject.type = 'Werebat';
```
Selecting elements and changing its text:
```javascript
const myHeading = document.querySelector('h1');
myHeading.textContent = 'Hello world!';
let playerName = 'Jane Doe (for now)';
const elPlayerName = document.getElementById('player-name');
elPlayerName.textContent = playerName;
```
Operators:
```javascript
const answer = 23 + 19;
let playerName = 'Francine';
let greeting = 'Welcome ' + playerName;
let arbitraryNumber = (9 - 3) * 8 + 9 / 3 * (23 + 2) / 2;
let isPlayerFrancine = playerName === 'Francine';
let truth = answer === 42;
let isFalse = 23 === 42;
let isTrue = 23 !== 42;
let alsoFalse = 42 === '42';
```
Conditionals:
```javascript
let iceCream = 'chocolate';
let players = 5;
if ( iceCream === 'chocolate' ) {
alert('Yay, I love chocolate ice cream!');
} else {
alert('Awwww, but chocolate is my favorite...');
}
if ( players >= 4 ) {
console.log('starting up the game engine');
}
```
Functions:
```javascript
// a function we already used:
alert ('Hiyaaaa!');
// another function we already used, which returns something useful:
let element = document.getElementById('player-name');
// a new function we create right now:
function multiply(num1,num2) {
let result = num1 * num2;
return result;
}
// using our new function
multiply(4, 7);
multiply(20, 20);
multiply(0.5, 3);
```
Some more advanced concepts are introduced in [Session III: Advanced Javascript, ES6 and beyond, jquery](https://tantemalkah.at/2021/artful-coding/slides/session3.html) of the mentioned course. In terms of web hacking you probably won't need most of them. But take a look, and also check out the reference materials linked below if you want to dig deeper into what you can do with Javascript.
References:
* [JavaScript — Dynamic client-side scripting](https://developer.mozilla.org/en-US/docs/Learn/JavaScript) @ MDN Web Docs
* [JavaScript Tutorial](https://www.w3schools.com/js/default.asp) @ W3Schools
* [Artful Coding 1: web-based games development](https://tantemalkah.at/artful-coding/2021wt/) (WT2021 @ dieAngewandte) - includes session 2 and 3 with quick intros on HTML, CSS, and JS, including slides and video recordings
## The Browser Environment
* Dev Tools
* Inspector
* 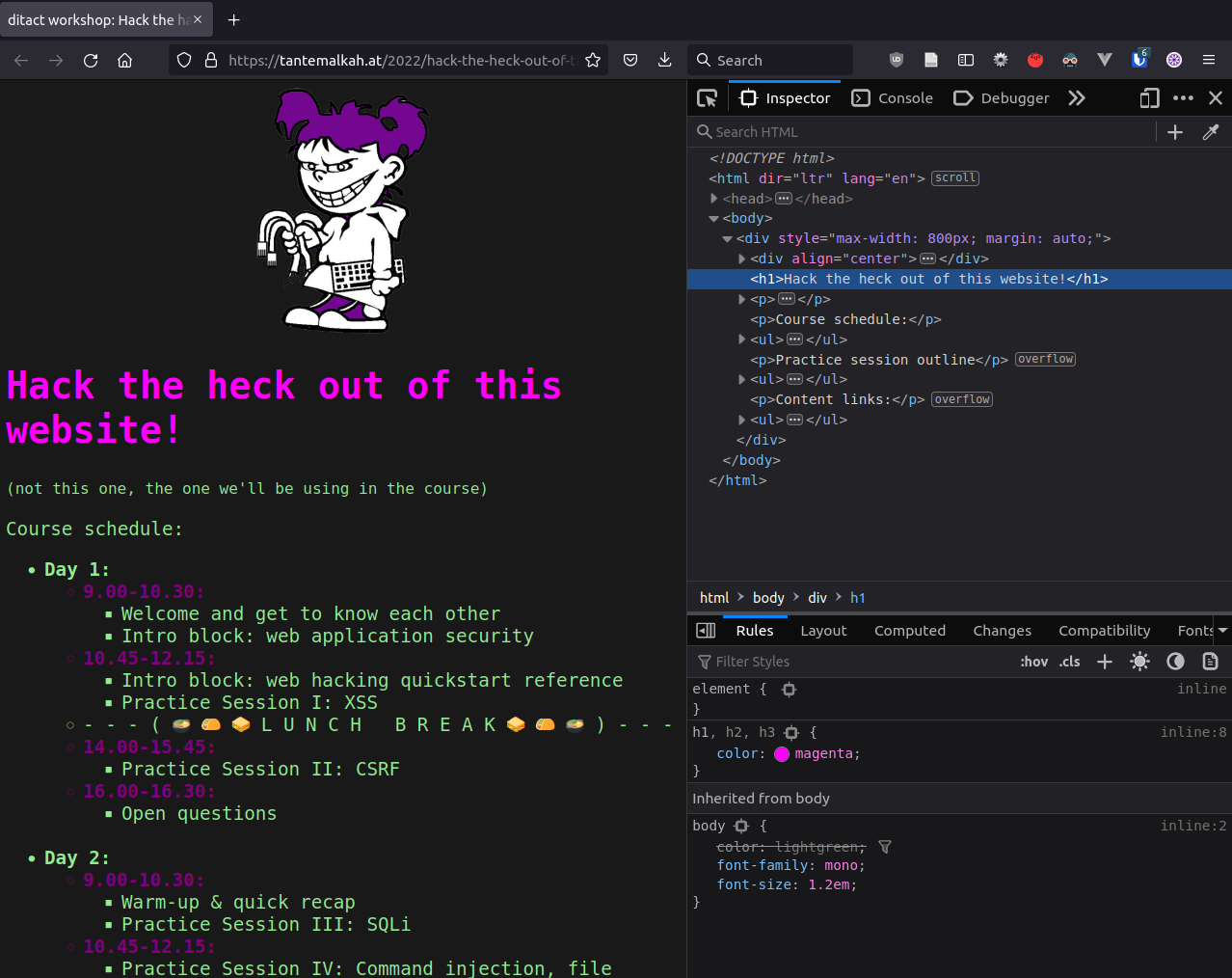
* Console
* 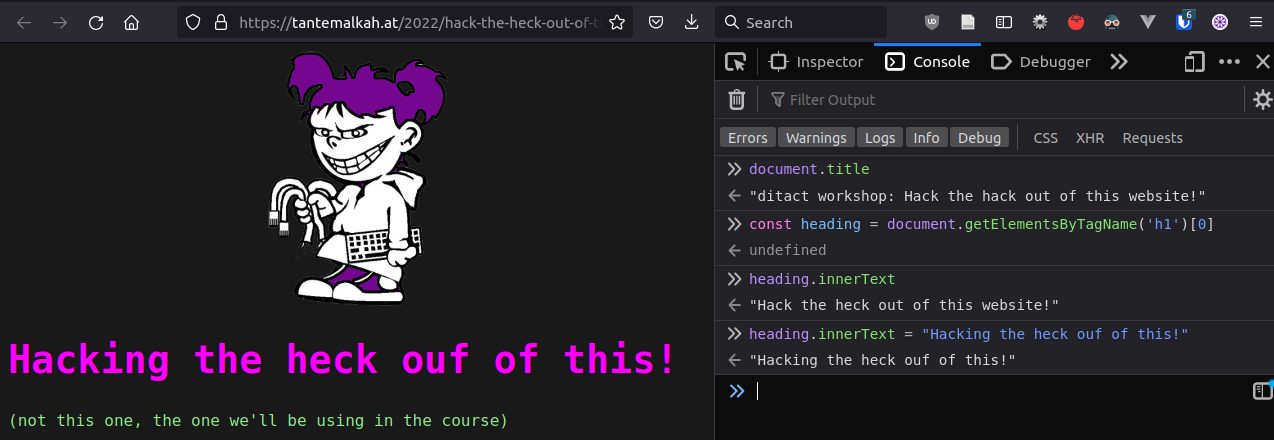
* Network
* 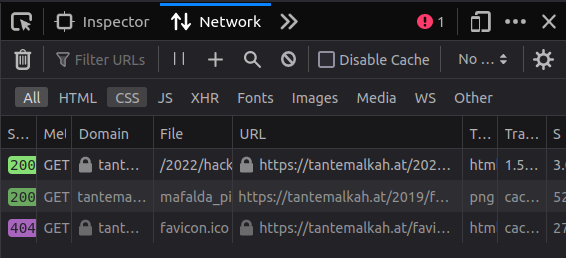
* Storage
* 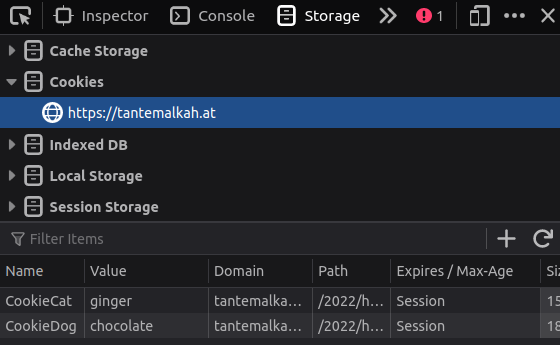
* Some useful commands:
* `alert("hey there!")`
* `document.body`
* `document.getElementById("someId")`
* `window.location`
* `window.onload`
* Clickable commands `onclick` in HTML tags:
* `<p onclick="alert('are you sure you should have clicked that?')">`
References:
* [Firefox DevTools User Docs](https://firefox-source-docs.mozilla.org/devtools-user/index.html)
* [Chrome DevTools](https://developer.chrome.com/docs/devtools/)
* [Introduction to the DOM](https://developer.mozilla.org/en-US/docs/Web/API/Document_Object_Model/Introduction) @ MDN Web Docs