# Use Godot First Time
###### tags: `Godot`
Extract some text, pictures as the note from Godot Engine documentation's [Step by step](https://docs.godotengine.org/en/stable/getting_started/step_by_step/index.html).
## [Object-oriented design and composition](https://docs.godotengine.org/en/stable/getting_started/introduction/godot_design_philosophy.html#object-oriented-design-and-composition)
## [Node](https://docs.godotengine.org/en/stable/getting_started/step_by_step/nodes_and_scenes.html#nodes)
All nodes have the following characteristics:
* A name.
* Editable properties.
* They receive callbacks to update every frame. => Time related.
* You can extend them with new properties and functions.
* You can add them to another node as a child.
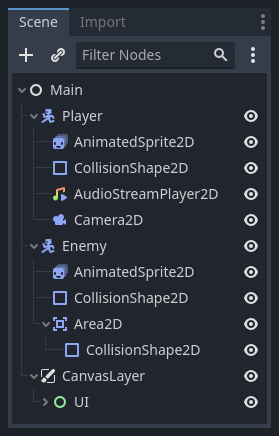
For example, a [CharacterBody2D](https://docs.godotengine.org/en/stable/classes/class_characterbody2d.html#class-characterbody2d) node, a [Sprite2D](https://docs.godotengine.org/en/stable/classes/class_sprite2d.html#class-sprite2d) node, a [Camera2D](https://docs.godotengine.org/en/stable/classes/class_camera2d.html#class-camera2d) node, and a [CollisionShape2D](https://docs.godotengine.org/en/stable/classes/class_collisionshape2d.html#class-collisionshape2d) node.
The node can cover another node, according to the placed order.
## [Scene](https://docs.godotengine.org/en/stable/getting_started/step_by_step/nodes_and_scenes.html#scenes)
Organizing nodes in a tree is called as constructing a scene. The Godot editor essentially is a scene editor. The engine only requires one as your application's **main scene**. This is the scene Godot will first load when you or a player runs the game.
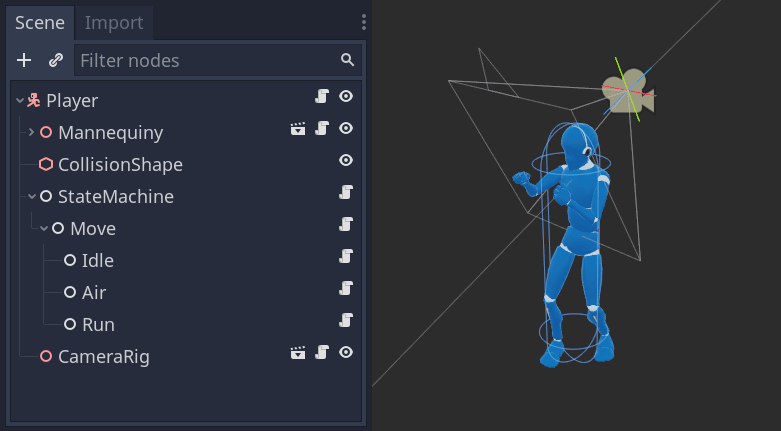
## Resource
Godot aggreagates the files as the resources and stores them in the filesystem, including: the pictures, scenes, scripts.

## [GDScript](https://docs.godotengine.org/en/stable/getting_started/step_by_step/scripting_languages.html#gdscript)
[GDScript](https://docs.godotengine.org/en/stable/tutorials/scripting/gdscript/gdscript_basics.html#doc-gdscript) is an object-oriented and imperative programming language built for Godot. Key features:
* Attach to node.
* Tight editor integration, with code completion for nodes, signals, and more information from the scene it's attached to.
* Built-in **vector** and transform types, making it efficient for heavy use of linear algebra, a must for games.
* Supports multiple threads as efficiently as statically typed languages.
* **No garbage collection**, as this feature eventually gets in the way when creating games. The engine counts references and manages the memory for you in most cases by default, but you can also control memory if you need to.
* Gradual typing. Variables have dynamic types by default, but you also can use type hints for strong type checks.
### `_init()`
The func keyword defines a new function named `_init`. This is a special name for our class's **constructor**. The engine calls `_init()` on every object or node upon creating it in memory, if you define this function.
### `_process(delta)`
Godot will call the function every frame and pass it an argument named **delta**, the time elapsed since the last frame.
### `_unhandled_input()`
* `_unhandled_input()` is a built-in virtual function that Godot calls every time the player presses a key.
* The [Input](https://docs.godotengine.org/en/stable/classes/class_inputevent.html#class-inputevent-method-is-action-pressed) singleton. A singleton is a globally accessible object. Godot provides access to several in scripts. It's the right tool to check for input every frame.
The [InputMap](https://docs.godotengine.org/en/stable/tutorials/inputs/input_examples.html#inputmap) is the most flexible way to handle a variety of inputs. You use this by creating named input actions, to which you can assign any number of input events, such as keypresses or mouse clicks. To see them, and to add your own, open Project -> Project Settings and select the InputMap tab. Click **Show Built-in Actions**:

### Signal
Other nodes can **connect** to the node's signal and call a function when the event occurs.
### [Vector math](https://docs.godotengine.org/en/stable/tutorials/math/vector_math.html#doc-vector-math)
Yes! It is the Vector! Velocity is a kind of vector.