# How to start programming with Python: a compilation of resources
## Why learn how to program?
> Learning to write programs stretches your mind, and helps you think better, creates a way of thinking things that I think is helpful in all domains.
> -Bill Gates,Microsoft.
> I think everybody in this country should learn how to program a computer...because it teaches you how to think.
> -Steve Jobs, Apple Inc.
> In fifteen years we'll be teaching programming just like reading and writing...and wondering why we didn't do it sooner.
> -Mark Zuckerberg, Facebook.
> Don't just play on your phone, program it.
> -Barack Obama, POTUS.
Technology, be it in the form of computers, phones, or anything in between, has become ubiquitous in our lives, and it will continue to shape our future. Logically, all the applications and advancements that make our lives more comfortable are fueled by the efforts of those behind the scenes, developers and engineers who work day-by-day to bring us a better product. Not only our reliance on these is going to keep increasing, but the job landscape in the next few decades will be in a state of constant change due to the automation of many jobs as artificial intelligence (AI) keeps phasing out entire industries.
However, computer-related occupations such as engineers and technicians working with computers, programmers and software developers are expected to keep growing and taking a bigger percentage of the market share. 7 out of the 10 best jobs in America for 2021, according to [Glassdoor](https://www.glassdoor.com/List/Best-Jobs-in-America-LST_KQ0,20.htm) involve programming.
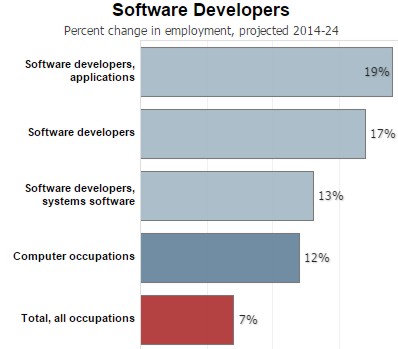
Added to this are all the endless applications of programming. George Gilder, an American investor, described computers as *"inscribing worlds on grains of sand"*. From mobile applications and webpages, to videogames, to intelligent assistants; all these are powered by the same core methodology: coding instructions for a computer to follow.
## Why programming in Python?
I wouldn't consider myself an expert programmer, not even close. But I have studied and used different programming languages during my life. Among these, roughly in order, are Visual Basic, Java, Arduino(C), MATLAB(R), Python, C++, HTML and CSS. By far the ones I'm the most comfortable are MATLAB and Python, with C++ taking a distant third.
I used MATLAB during most of my undergraduate studies, since that's what we were provided and "taught" in our engineering classes. However, the proprietary nature of MATLAB, made it difficult to use after graduation since the licenses were expensive, and at times it could feel more like a calculator on steroids rather than a fully developed, multi-purpose programming language.
During my last semester of undergraduate, I took a course in Python and since then I haven't looked back. I will briefly try to summarize the main reasons why I would recommend Python to beginner and novice programmers, and most people planning to do work on scientific computing, including but not limited to data science, machine learning, deep learning, computer vision, natural language processing and others.
### Reason No. 1: ease of syntax
It's extremely easy to get started with due to its natural, human-like syntax that includes no variable type declaration, among others that make it easier for both beginners and seasoned programmers to read and write. See the comparison below for the most basic program to start with *Hello, world!* written in C++, Java, and Python:
```cpp=
// C++
#include <iostream>
int main()
{
std::cout << "Hello, world!\n";
return 0;
}
```
```java=
// Java
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
```
```python=
# Python
print("Hello, world!")
```
### Reason No. 2: easy to setup and start with
Python usually comes pre-installed on MacOS and Linux, and it's easy to install on windows. There's also many alternatives online including [Coding Ground Python Online Interpreter](https://www.tutorialspoint.com/execute_python3_online.php), [Google Colab](https://colab.research.google.com/), [Kaggle Kernels](https://www.kaggle.com/kernels), and [others](https://www.dataschool.io/cloud-services-for-jupyter-notebook/).
### Reason No. 3: interactive visualization using IPython and Jupyter Notebooks
A picture is worth a thousand words. Below you can see examples of how IPython/Jupyter Notebooks make it easier to execute and visualize what you're coding.
<figure>
<img src="https://2.bp.blogspot.com/-kXIJLgqGmD4/XNLxsIz3eVI/AAAAAAAAAxg/T-ANzWYq8yoI1hx5FwwgishoVe1qtenYwCLcBGAs/s1600/notebookex1.PNG" alt="IPython/Jupyter Notebooks for Data Visualization">
<figcaption style="align: left; text-align:center;"><a href="https://projectcodeed.blogspot.com/2019/08/setting-up-jupyter-notebooks-for-data.html">IPython/Jupyter Notebooks</a></figcaption>
</figure>
<figure>
<img src="https://arogozhnikov.github.io/images/jupyter/example-notebook.png" alt="IPython/Jupyter Notebooks for Data Visualization">
<figcaption style="align: left; text-align:center;"><a href="https://arogozhnikov.github.io/2016/09/10/jupyter-features.html">IPython/Jupyter Notebooks</a></figcaption>
</figure>
### Reason No. 4: active community with thousands of open-source libraries
This combined with abstraction and high-level APIs allow for developers to build powerful applications in relatively few lines of code, without as much understanding of what's happening below the hood, compared to others. Note: I still think it's good to understand what's happening but it's not always necessary.
I already gave a few examples above on how we can in just a few lines obtain visualizations, but we can also even setup machine learning models (in this case for image classification) in a similar fashion:
```python=
from imageai.Classification import ImageClassification
import os
execution_path = os.getcwd()
prediction = ImageClassification()
prediction.setModelTypeAsResNet50()
prediction.setModelPath( execution_path + "\resnet50_imagenet_tf.2.0.h5")
prediction.loadModel()
predictions, percentage_probabilities = prediction.classifyImage("C:\Users\MyUser\Downloads\sample.jpg", result_count=5)
for index in range(len(predictions)):
print(predictions[index] , " : " , percentage_probabilities[index])
```
<figure>
<img src="https://i.imgur.com/oCbTXLm.jpg" alt="IPython/Jupyter Notebooks for Data Visualization">
<figcaption style="align: left; text-align:center;"><a href="https://guymodscientist.medium.com/image-prediction-with-10-lines-of-code-3266f4039c7a">Example of how high-level APIs allow us to obtain great results with only a few lines of code</a></figcaption>
</figure>
### Alternative programming languages
Other possible languages to learn include C, C++, Java, Javascript, but I would still recommend Python for starters, and then based on your goals you can learn another. After all, programming is all about learning and keeping up as technologies further change and develop.
<figure>
<img src="https://i.imgur.com/sqv9duJ.png" alt="Projections for major programming languages">
<figcaption style="align: left; text-align:center;">Projections for major programming languages based on number of questions on <a href="https://stackoverflow.blog/2017/09/06/incredible-growth-python/">Stack Overflow</a></figcaption>
</figure>
### Use cases for Python
* Web and Internet Development: many popular websites including Instagram, Google, Spotify, Netflix, Uber, Dropbox, Reddit, among others, were [built using Python](https://learn.onemonth.com/10-famous-websites-built-using-python/).
* Scientific and Numeric: mathematics, science, engineering, data analysis, machine learning and related.
* Scripting: automation of mundane, simple tasks. For example filling out internet (or any) forms, retrieving content from the internet (scraping), organizing files, etc.
<figure>
<img src="https://2.bp.blogspot.com/-oeOzu13C26U/V1_2uXbFE4I/AAAAAAAAALI/2RmiWjCb--YUVO6MAg3pG5eIOISFkVwBgCLcB/s1600/custom-web-scraping-624x301.png" alt="Python for Web Data Scraping">
<figcaption style="align: left; text-align:center;"><a href="https://www.datacamp.com/community/tutorials/making-web-crawlers-scrapy-python">Python for Web Data Scraping</a></figcaption>
</figure>
<figure>
<img src="https://cdn-images-1.medium.com/max/800/1*JxbqIQmD_E3M3I7Tjo0OqA.jpeg" alt="Python for Data Visualization">
<figcaption style="align: left; text-align:center;"><a href="https://www.kdnuggets.com/2018/07/5-quick-easy-data-visualizations-python-code.html">Python for Data Visualization</a></figcaption>
</figure>
<figure>
<img src="https://scipy-lectures.org/_images/random_c.jpg" alt="Python for Scientific Computing">
<figcaption style="align: left; text-align:center;"><a href="https://scipy-lectures.org/intro/intro.html">Python for Scientific Computing</a></figcaption>
</figure>
<figure>
<img src="https://github.com/thtang/CheXNet-with-localization/raw/master/output/bb_select.JPG" alt="Python for Medical Image Analysis">
<figcaption style="align: left; text-align:center;"><a href="https://github.com/thtang/CheXNet-with-localization">Python for Medical Image Analysis</a></figcaption>
</figure>
<figure>
<img src="https://i.imgur.com/Kycx4eZ.jpg" alt="Python for Agriculture">
<figcaption style="align: left; text-align:center;"><a href="https://arxiv.org/abs/1907.11819">Python for Agriculture</a></figcaption>
</figure>
## Basics of Python
[Automate the Boring Stuff with Python](https://automatetheboringstuff.com/) by Al Sweigart. It's, in my opinion, the best book to get started with programming. It covers the basics in a really intuitive way, and then goes through a number of possible simple use cases for scripting and automation of tasks. It's available in a text format in the provided website, as a hard-copy version, a [Udemy online course](https://www.udemy.com/course/automate/?couponCode=FEB2021FREE), and a [YouTube playlist](https://www.youtube.com/watch?v=Z9IxxW7428A&list=PL0-84-yl1fUnRuXGFe_F7qSH1LEnn9LkW&index=15&ab_channel=AlSweigart). The first 7 chapters (starting from 0, so 0 to 6) are absolutely essential to understand the basics of the languages and I would recommend them to anyone that's getting started to grasp the fundamentals of programming with Python. From there the rest of the book covers different automation tasks, so it's skippable but still pretty interesting and can be good practice depending on your goals.
Another one that is widely acclaimed as a great starter book is [Python Crash Course](https://nostarch.com/pythoncrashcourse2e) by Eric Matthes. This one is available to purchase as a hard-copy book, e-book available in that website, but the [PDF](http://bedford-computing.co.uk/learning/wp-content/uploads/2015/10/No.Starch.Python.Oct_.2015.ISBN_.1593276036.pdf) can also be found online. To be honest I have never read it, but I deeply agree with his views on coding:
> Code is power, and learning to code gives you power. The more you understand code, the more you understand how much impact tech platforms and products have on people's lives - whether through the implementation of specific features, or the lack of implementation of certain features. When you've built a platform that a significant part of society uses for communication, for example, your code has direct impact on society itself. When you write code that helps determine who gets a loan, you impact who can afford to buy a home.
>
> Those who have power are desperate to hold on to that power, and are willing to use force to hold on to that power.
>
> As you learn to code, please be aware of the power you are gaining. As you work on projects, whether your own projects or those that are controlled by someone else, please focus on projects that share power. Please refuse to work on projects that consolidate power, especially for those who will use it against others.
> -[Eric Matthes](https://ehmatthes.github.io/pcc_2e/), Coding is political.
Asides from this there's many online courses in [Udemy](https://www.udemy.com/topic/python/), [Coursera](https://www.coursera.org/courses?query=python), [edX](https://www.edx.org/learn/python) and YouTube, with special shoutout to the ones from [FreeCodeCamp](https://www.youtube.com/watch?v=rfscVS0vtbw&ab_channel=freeCodeCamp.org) and [Corey Schafer](https://www.youtube.com/watch?v=YYXdXT2l-Gg&list=PL-osiE80TeTskrapNbzXhwoFUiLCjGgY7&ab_channel=CoreySchafer). In the end we all have different preferences when it comes to learning, so a possibility is checking out a few minutes of each course to get a feel of the instructor style and the course structure, and go with one that you like. After all, this is just the beginning.
### Basics of Computer Science
Simultaneously while learning programming in Python, it may be good to get a basic understanding of Computer Science, how do computers work and why they work the way they do. This, and much more, is what you will learn in [edX CS50x: Introduction to Computer Science](https://www.edx.org/course/cs50s-introduction-to-computer-science) with Professor David J. Malan, from Harvard University. The first few lectures of this course are hands down one of the best educational experiences I've had in my life. Some of the topics included in the course that are fundamental for anyone taking programming seriously include abstraction, algorithms and data structures, among many others.
## What comes next?
At this point you should be familiar with the following basic concepts: variables, flow control (ifs and loops), basic data types (ints, floats, string, bools) and data structures (lists, sets, dictionaries), functions and ideally classes. Classes are not covered in Automate the Boring but here's a good [playlist](https://youtu.be/ZDa-Z5JzLYM) on the topic.
What comes later? Well you could take more courses in more specific applications, for which I may (see section on [Python for scientific computing](#Python-for-scientific-computing)) or may not have specific recommendations. Something I definitely recommend though, for everyone who has gotten to this point, is to read or watch at least a bit about **version control** and why it's important for anything software related. I personally think the following playlist is one of the best regarding the topic: [Git, GitHub and Version Control Playlist from *The Net Ninja*](https://www.youtube.com/watch?v=3RjQznt-8kE&list=PL4cUxeGkcC9goXbgTDQ0n_4TBzOO0ocPR&ab_channel=TheNetNinja)
To be honest, at this point, the best thing I would recommend doing though, is to look for something, something where you could apply what you've learned, even if you don't know all the details, because I've found that most people learn the most by doing. You can read and sit through lectures all you want, but it's not until you have sitten through an end-to-end project, that you really get to connect all those dots of everything you've learned. And usually during the process you also end up learning quite a lot of new things. So go there and build (or break) some program!
### Coding, algorithmic thinking and problem solving
In my opinion, programming is much more about than knowing a language specific syntax, or remembering which functions or library to use for what. Rather, programming is knowing how to think *algorithmically*; this is, how to break a big problem into smaller problems you can solve in a methodological fashion.
> "Select multiple columns of array python"
> -Common search I often do while working with data
It's normal to feel lost. Sometimes it can help to write your ideas, draw them, or discuss them with others. Also, if you don't know how to do something specific, as long as you can express it in the correct, concise way many times you can just Google it! For me, one of the most important parts of programming is understanding how to break a big problem into those small, solvable problems that you can Google, then put everything together.

### Python for scientific computing
If you're already familiar with Python, or with programming in general, but want to begin getting into scientific computing, then this is probably in my opinion one of the quickest tutorials to learn the basics: [Stanford CS231n Python Numpy Tutorial (with Jupyter and Colab)](https://cs231n.github.io/python-numpy-tutorial/). It includes three important libraries for scientific computing: NumPy, for linear algebra, SciPy for scientific and engineering applications, and Matplotlib, for plotting. If you come from a MATLAB background this tutorial may also be good: [NumPy for MATLAB users](https://numpy.org/doc/stable/user/numpy-for-matlab-users.html).
## Conclusion
This post provides a list of resources for people looking to get started into programming, focusing on Python.
If you like this post, or have any questions, feel free to leave a comment or contact me on any of my socials, found at the bottom of my [Github Pages](https://arkel23.github.io/).