226.Invert Binary Tree
===
###### tags: `Easy`,`Tree`,`BFS`,`DFS`,`Binary Tree`
[226. Invert Binary Tree](https://leetcode.com/problems/invert-binary-tree/)
### 題目描述
Given the `root` of a binary tree, invert the tree, and return its root.
### 範例
**Example 1:**
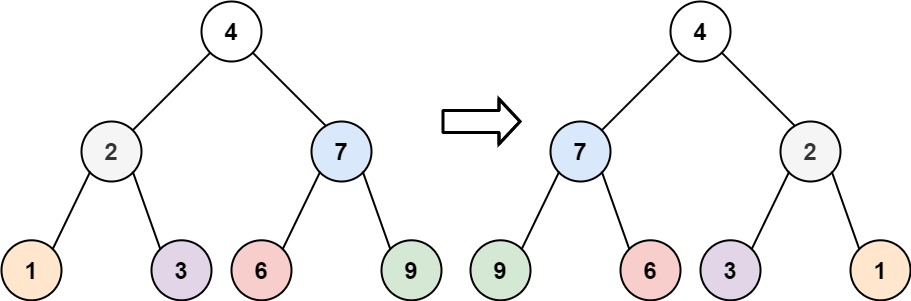
```
Input: root = [4,2,7,1,3,6,9]
Output: [4,7,2,9,6,3,1]
```
**Example 2:**
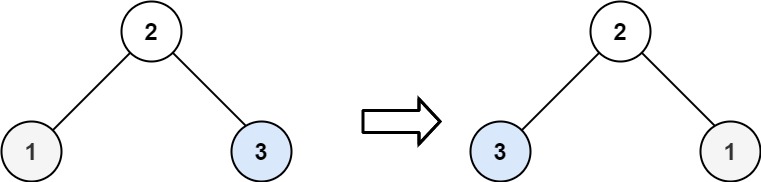
```
Input: root = [2,1,3]
Output: [2,3,1]
```
**Example 3:**
```
Input: root = []
Output: []
```
**Constraints**:
* The number of nodes in the tree is in the range `[0, 100]`.
* -100 <= `Node.val` <= 100
### 解答
#### Python
```python=
class Solution:
def invertTree(self, root: Optional[TreeNode]) -> Optional[TreeNode]:
if not root: return
root.left, root.right = self.invertTree(root.right), self.invertTree(root.left)
return root
```
> [name=Ron Chen][time=Sat, Feb 18, 2023]
#### Javascript
```javascript=
function invertTree(root) {
if (root === null) return null;
const queue = [root];
while (queue.length) {
const node = queue.shift();
const temp = node.left;
node.left = node.right;
node.right = temp;
if (node.left) queue.push(node.left);
if (node.right) queue.push(node.right);
}
return root;
}
```
> [name=Marsgoat][time=Feb 20, 2023]
### Reference
https://twitter.com/mxcl/status/608682016205344768
[回到題目列表](https://hackmd.io/@Marsgoat/leetcode_every_day)