129.Sum Root to Leaf Numbers
===
###### tags: `Medium`,`Tree`,`DFS`
[129. Sum Root to Leaf Numbers](https://leetcode.com/problems/sum-root-to-leaf-numbers/)
### 題目描述
You are given the `root` of a binary tree containing digits from `0` to `9` only.
Each root-to-leaf path in the tree represents a number.
* For example, the root-to-leaf path 1 -> 2 -> 3 represents the number 123.
Return *the total sum of all root-to-leaf numbers. Test cases are generated so that the answer will fit in a **32-bit** integer.*
A **leaf** node is a node with no children.
### 範例
**Example 1:**
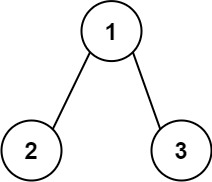
```
Input: root = [1,2,3]
Output: 25
Explanation:
The root-to-leaf path 1->2 represents the number 12.
The root-to-leaf path 1->3 represents the number 13.
Therefore, sum = 12 + 13 = 25.
```
**Example 2:**
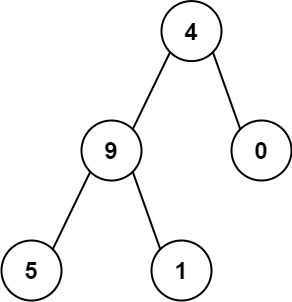
```
Input: root = [4,9,0,5,1]
Output: 1026
Explanation:
The root-to-leaf path 4->9->5 represents the number 495.
The root-to-leaf path 4->9->1 represents the number 491.
The root-to-leaf path 4->0 represents the number 40.
Therefore, sum = 495 + 491 + 40 = 1026.
```
**Constraints**:
* The number of nodes in the tree is in the range `[1, 1000]`.
* 0 <=` Node.val` <= 9
* The depth of the tree will not exceed `10`.
### 解答
#### Python
```python=
class Solution:
def sumNumbers(self, root: Optional[TreeNode]) -> int:
nums = []
def dfs(root, num=None):
if not root: return
if not num: num = []
num.append(str(root.val))
if not root.left and not root.right:
num_str = "".join(num)
nums.append(int(num_str))
dfs(root.left, num)
dfs(root.right, num)
num.pop()
dfs(root)
return sum(nums)
```
> [name=Ron Chen][time=Tue, Mar 14, 2023]
### Reference
[回到題目列表](https://hackmd.io/@Marsgoat/leetcode_every_day)