104.Maximum Depth of Binary Tree
===
###### tags: `Easy`,`Tree`,`BFS`,`DFS`,`Binary Tree`
[104. Maximum Depth of Binary Tree](https://leetcode.com/problems/maximum-depth-of-binary-tree/)
### 題目描述
Given the `root` of a binary tree, return *its maximum depth.*
A binary tree's **maximum depth** is the number of nodes along the longest path from the root node down to the farthest leaf node.
### 範例
**Example 1:**
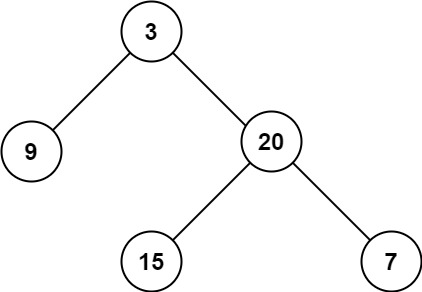
```
Input: root = [3,9,20,null,null,15,7]
Output: 3
```
**Example 2:**
```
Input: root = [1,null,2]
Output: 2
```
**Constraints**:
* The number of nodes in the tree is in the range [0, 10^4^].
* -100 <= `Node.val` <= 100
### 解答
#### Python
```python=
class Solution:
def maxDepth(self, root: Optional[TreeNode]) -> int:
return max(self.maxDepth(root.left), self.maxDepth(root.right)) + 1 if root else 0
```
> [name=Ron Chen][time=Thu, Feb 16, 2023]
#### Javascript
```javascript=
function maxDepth(root) {
if (root === null) return 0;
return Math.max(maxDepth(root.left), maxDepth(root.right)) + 1;
}
```
非遞迴版本
```javascript=
function maxDepth2(root) {
if (root === null) return 0;
const queue = [root];
let depth = 0;
while (queue.length > 0) {
const size = queue.length;
for (let i = 0; i < size; i++) {
const node = queue.shift();
if (node.left !== null) queue.push(node.left);
if (node.right !== null) queue.push(node.right);
}
depth++;
}
return depth;
}
```
> [name=Marsgoat][time=Thu, Feb 16, 2023]
#### C#
```csharp=
public class Solution {
public int MaxDepth(TreeNode root) {
return root == null ? 0 : 1 + Math.Max(MaxDepth(root.left), MaxDepth(root.right));
}
}
```
> [name=Jim][time=Thu, Feb 16, 2023]
### Reference
[回到題目列表](https://hackmd.io/@Marsgoat/leetcode_every_day)